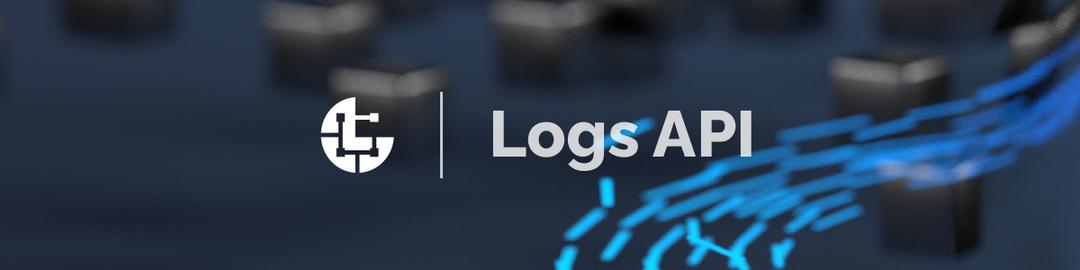
Overview
Logs API is a solution that allows the user to gather data on interactions with smart contracts by analyzing their log events, which can be decoded and converted into a human-readable format when a smart contract's ABI is provided.
A successful request includes information on topics, log data, transaction hash, block hash, block number,
timestamp, EVM opcode, origin, and contract. When making a request, it's mandatory to specify either topic_0
,
topic_1
, topic_2
, topic_3
, contract
, or origin
. The range should be defined by either block_number
,
timestamp
, or block_hash
.
Endpoint
Get LogsGET, POST
Retrieve data on log events by specifying ranges and other query parameters; the data can be decodedGet CSV Export LogsGET
Retrieve data on log events in comma-separated values by specifying ranges and other query parametersAvailability
Blockchain Network | Availability |
---|---|
Ethereum Mainnet | ✅ |
BNB Smart Chain Mainnet | ✅ |
Polygon PoS | ✅ |
Polygon zkEVM | ✅ |
Avalanche C-Chain | ✅ |
Arbitrum One | ✅ |
Metis Andromeda | ✅ |
Ethereum Sepolia | ✅ |
Ethereum Holešky | ✅ |
BNB Smart Chain Testnet | ✅ |
opBNB Testnet | ✅ |
Arbitrum Sepolia | ✅ |
Polygon Amoy | ✅ |